How to set Tkinter Window Background Color?
Set Tkinter Window Background Color
The default background color of a GUI with Tkinter is grey. You can change that to any color based on your application's requirement.
In this tutorial, we will learn how to change the background color of Tkinter window.
There are two ways through which you can change the background color of window in Tkinter. They are:
- Using
configure(bg='')
method of tkinter.Tk class. or - Directly setting the
bg
property of tkinter.Tk.
In both of the above said cases, set bg property with a valid color value. You can either provide a valid color name or a 6-digit hex value with # preceding the value, as a string.
Examples
In the following examples, we shall learn how to change the background color of a Tkinter window.
1. Change background color using configure method
In this example, we will change the Tkinter window's background color to blue.
Python Program
from tkinter import *
gui = Tk(className='Python Examples - Window Color')
# set window size
gui.geometry("400x200")
#set window color
gui.configure(bg='blue')
gui.mainloop()
Output in Windows
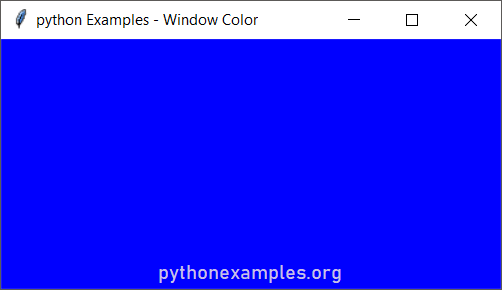
Output in macOS
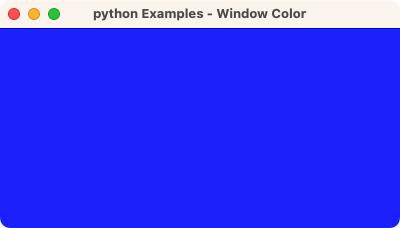
2. Change background color using bg property
In this example, we will change the Tkinter window's background color to blue.
Python Program
from tkinter import *
gui = Tk(className='Python Examples - Window Color')
# set window size
gui.geometry("400x200")
#set window color
gui['bg']='green'
gui.mainloop()
Output in Windows
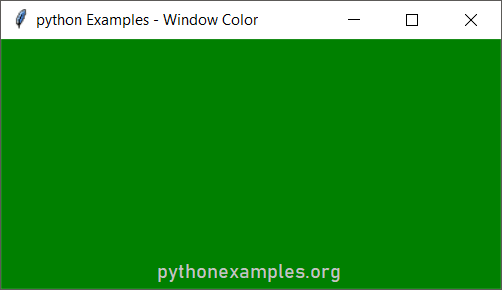
Output in macOS
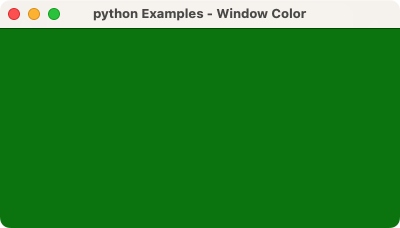
3. Change background color by using background for bg
You can use the property name bg
as in the above two examples, or also use the full form background
. In this example, we will use the full form background
to set the background color of Tkinter window.
Python Program
from tkinter import *
gui = Tk(className='Python Examples - Window Color')
# set window size
gui.geometry("400x200")
#set window color
gui['background']='yellow'
gui.mainloop()
Output in Windows
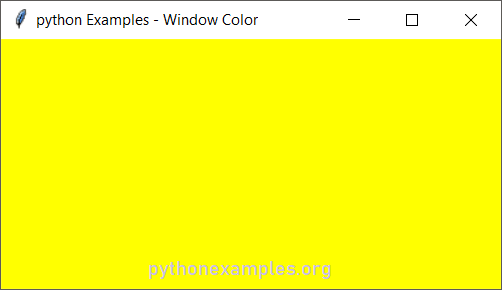
Output in macOS
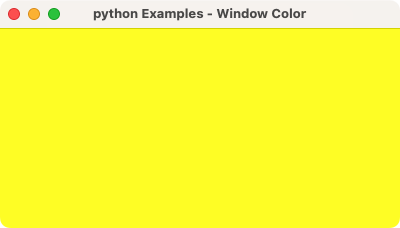
4. Using HEX code for background color
As already mentioned during the start, you can provide a HEX equivalent value for a color. In this example, we provide a HEX value to color and check the output.
Python Program
from tkinter import *
gui = Tk(className='Python Examples - Window Color')
# set window size
gui.geometry("400x200")
#set window color
gui['background']='#856ff8'
gui.mainloop()
Output in Windows
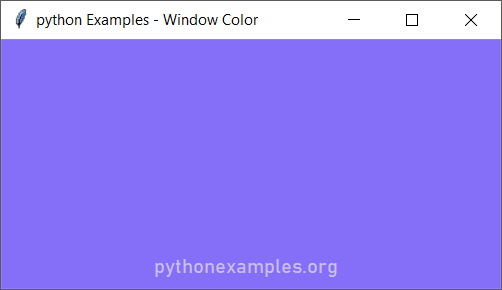
Output in macOS
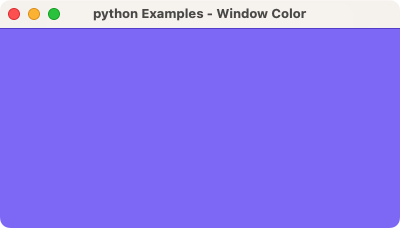
Summary
In this tutorial of Python Examples, we learned how to change the background color of Tkinter GUI window, with the help of well detailed Python example programs.