Tkinter Label - On Click Event
Tkinter Label - On click event
In Tkinter, you can bind a click event to a Label widget using the bind()
method.
To execute a function when user clicks on a Label widget in Tkinter, create a Label widget and define a callback function, then bind the mouse left-click event to the label object with the event handler function.
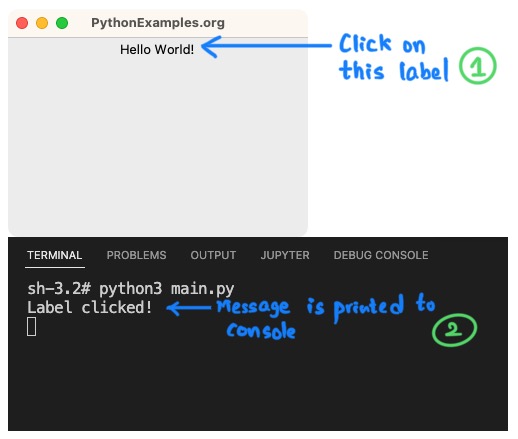
The syntax to bind the mouse left-click event to the label object to execute a function label_clicked is shown in the following.
label.bind("<Button-1>", label_clicked)
where <Button-1> specifies the mouse left-click event.
In this tutorial, you will learn how to set a event handler function for mouse click event on a Label widget in Tkinter, with examples.
Examples
1. Print a message when Label widget is clicked
In this example, we shall create a Label widget label with some text, and bind this label object to a mouse left-click event with a function label_clicked. In the callback function, we shall print the message Label clicked!.
Program
import tkinter as tk
# Event handler function for the label click
def label_clicked(event):
print("Label clicked!")
# Create the main window
window = tk.Tk()
window.title("PythonExamples.org")
window.geometry("300x200")
# Create a label widget
label = tk.Label(window, text="Hello World!")
# Bind the click event to the label
label.bind("<Button-1>", label_clicked)
# Pack the label widget to display it
label.pack()
# Run the application
window.mainloop()
Output
Run on Windows 10.
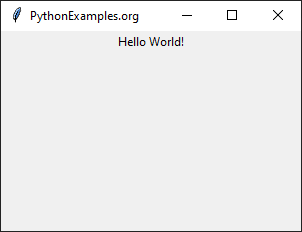
Run on MacOS.
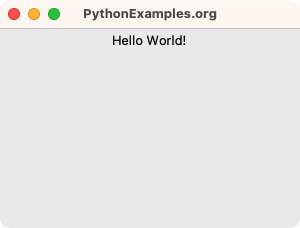
Click on the Hello World! label.
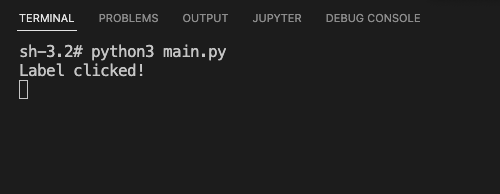
Summary
In this Python Tkinter tutorial, we learned how to execute a function when user clicks on a Label widget, with examples.