Python Tkinter Tutorial
Tkinter
Tkinter is a standard Python library used for creating graphical user interfaces (GUIs). It provides a set of tools and widgets that allow developers to build interactive applications with buttons, labels, entry fields, menus, and more.
In the following sections, we cover basics of Tkinter, widgets, etc.
Tkinter Window
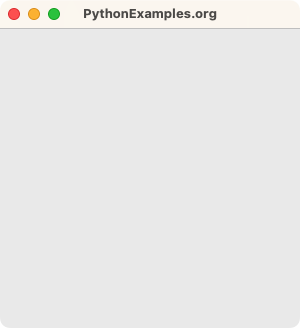
Window Basics
- Tkinter - Window example
- Tkinter - Set title for window
- Tkinter - Set window size
- Tkinter - Resizable window
- Tkinter - Non-resizable window
Window Styling
Events
- Tkinter - Window resize event
- Tkinter - Refresh window
- Tkinter - Bind Escape key to close window
- Tkinter - Close window programmatically
- Tkinter - Maximized window
Tkinter Label
The Label
widget in Tkinter is used to display text or images on a graphical user interface (GUI). It provides a way to present static content or information to the user.
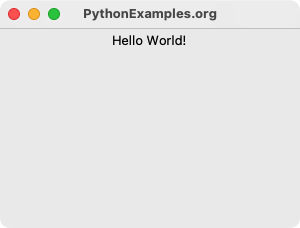
The following tutorials cover the creation of labels, styling of labels, and some event handling techniques on labels.
Basics
Styling
- Tkinter Label - Font size
- Tkinter Label - Italic text
- Tkinter Label - Underline text
- Tkinter Label - Bold text
- Tkinter Label - Background color
- Tkinter Label - Foreground color
- Tkinter Label - Width and height
- Tkinter Label - Border
- Tkinter Label - Center align text
- Tkinter Label - Left align text
- Tkinter Label - Right align text
- Tkinter Label - Background image
Events
Tkinter Button
In Tkinter, a button is a graphical user interface (GUI) widget that allows users to interact with your application by clicking on it.
A button typically represents an action or a command that can be triggered when clicked.
Button Basics
Button Styling
- Tkinter Button – Change font family, font size, and style
- Tkinter Button - Background color
- Tkinter Button - Change color on mouse click
Button Events
Tkinter Entry
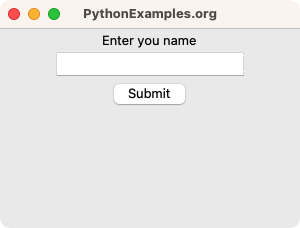
Entry widget basics
- Tkinter Entry
- Tkinter Entry - Password
- Tkinter Entry - Get the input value
- Tkinter Entry - Insert text
- Tkinter Entry - Delete value
- Tkinter Entry - Disable
- Tkinter Entry - Placeholder
- Tkinter Entry - Default text
- Tkinter Entry - Validation
Entry Widget Styling
- Tkinter Entry - Background color
- Tkinter Entry - Text color
- Tkinter Entry - Font size
- Tkinter - Left align text in Entry widget
- Tkinter - Right align text in Entry widget
- Tkinter - Center align text in Entry widget
- Tkinter Entry - Set width
Entry widget events
- Tkinter Entry - Handle Focus In and Focus Out events
- Tkinter Entry - On enter key event
- Tkinter Entry - On Enter/Return key focus next widget
- Tkinter Entry - On change
Tkinter Checkbutton
Checkbutton widget is used to allow users to select or deselect options.
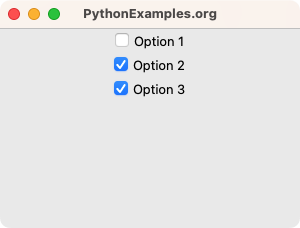
The following tutorials cover the topics of how a Checkbutton is created, options of a Checkbutton, how to handle the events related to this widget, and more.
- Tkinter Checkbutton
- Tkinter Checkbutton() Options
- Tkinter Checkbutton - Command
- Tkinter - Create Checkbutton widgets from a dictionary
- Tkinter Checkbutton - Checked by default
- Tkinter Checkbutton - Disable
- Tkinter Checkbutton - Get selected values
- Tkinter Checkbutton - Set value
- Tkinter Checkbutton - Check if selected
- Tkinter Checkbutton - On change event
Tkinter Menu Widget
In Tkinter, Menus provide a way to create hierarchical navigation or actions in Tkinter-based applications. Menus offer a convenient way to organize and present various commands and options to the user.
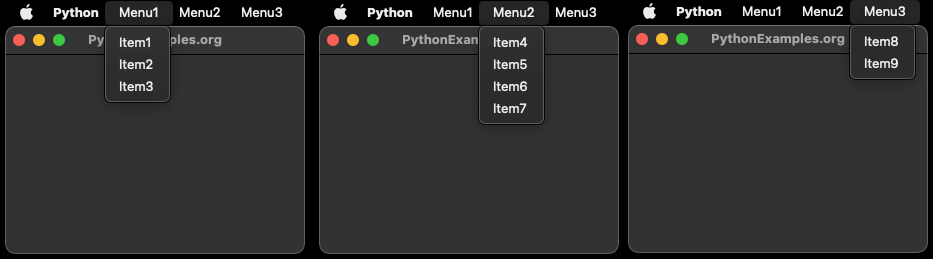
The following tutorials covert topics related to the Menu widget in Tkinter.
Menu Widget Basics
- Tkinter - Create Menu bar
- Tkinter - Create Menu
- Tkinter - Add separator in Menu
- Tkinter - Create submenus
- Tkinter - Checkbutton Menu items
- Tkinter - Radiobutton Menu items
- Tkinter - Add keyboard shortcut to menu item
- Tkinter - Disable menu item
- Tkinter - Tear-off Menu (Popup menus)
Menu Events and Actions
- Tkinter - Command callback function for Menu item
- Tkinter - Pass arguments in the command function on menu item click event
- Tkinter - Add icon to Menu item
- Tkinter - Context Menus (Popup Menus)
Advanced Menu Tutorials
Tkinter Canvas
Canvas widget is used to draw and manipulate graphics, such as lines, shapes, text, and images, on a rectangular area.
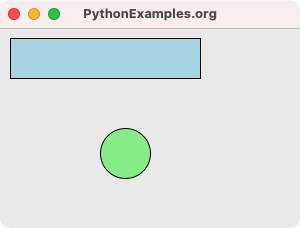
Tkinter Frame
Frame is a container widget that acts as a rectangular region to hold other widgets or groups of widgets.
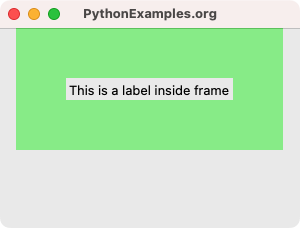
Tkinter Listbox
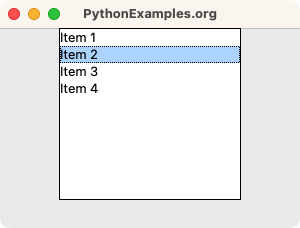
Tkinter Message-box
In Tkinter, a messagebox is a pre-defined dialog box that provides a simple way to display messages or prompts to the user.
It is commonly used to show information, warnings, errors, or to ask for confirmation from the user.
- Tkinter messagebox
- Tkinter messagebox - Example
- Tkinter messagebox - Show info
- Tkinter messagebox - Show warning
- Tkinter messagebox - Show error
- Tkinter messagebox - Ask Yes or No
- Tkinter messagebox - Ask OK or Cancel
- Tkinter messagebox - Ask Yes, No, or Cancel
- Tkinter messagebox - Ask Retry or Cancel
- Tkinter messagebox - Without buttons
- Tkinter messagebox - showinfo() return value
Tkinter Dialogs
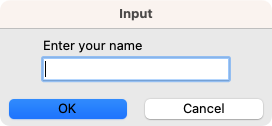
Standard Tkinter input dialogs
File selection dialogs
Tkinter Radiobutton
Radiobutton widget is used to display a radio button. We can use a group of radio buttons to select a single option from a set of mutually exclusive options.
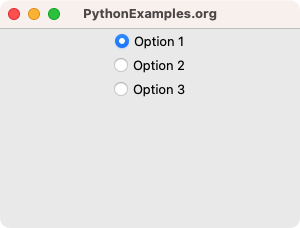
Basics
- Tkinter Radiobutton
- Tkinter Radiobutton - Groups
- Tkinter Radiobutton - Clear selection
- Tkinter Radiobutton - Default selection
- Tkinter Radiobutton - Get selected value
- Tkinter Radiobutton - Check if selected
- Tkinter Radiobutton - Select an option programmatically
Radiobutton events
Tkinter Scale
The Scale widget in Tkinter is used to create a slider control that allows the user to select a value from a range.
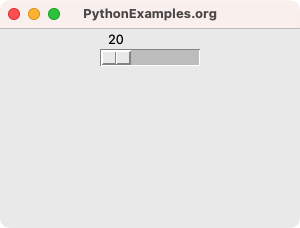