Select a radio button by value in Selenium - Python Examples
Selenium Python - Select a radio button by value
In this tutorial, you will learn how to select a radio button by value in value attribute, in Selenium Python.
To select a radio button by value in Selenium Python, form the XPath expression that the element has the following two attribute value.
- type="radio"
- value="your_value"
The XPath expression would be as shown in the following.
"//input[@name='x' and @value='your_value']"
You can use this XPath expression with find_element()
method of driver object, and find the radio button with a specific value for value attribute.
radio_button = driver.find_element("//input[@name='x' and @value='your_value']")
After finding the radio button, call click()
method on the radio button WebElement object.
radio_button.click()
Example
In this example, we shall consider loading the webpage at URL: /tmp/selenium/index-16.html. The contents of this HTML file is given below.
The webpage has a radio group for selecting a favorite subject.
<html>
<body>
<h3>My Favourite Subject</h3>
<form action="">
<input type="radio" id="physics" name="fav_subject" value="Physics">
<label for="physics">Physics</label><br>
<input type="radio" id="mathematics" name="fav_subject" value="Mathematics">
<label for="mathematics">Mathematics</label><br>
<input type="radio" id="chemistry" name="fav_subject" value="Chemistry">
<label for="chemistry">Chemistry</label>
</form>
</body>
</html>
In the following program, we initialize a webdriver, navigate to a specified URL, get the radio button with value equal to "Chemisty"
, and call click()
method on the radio button element.
We shall take screenshots before and after selecting the radio button.
Python Program
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
# Setup chrome driver
service = ChromeService(executable_path=ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
driver.set_window_size(500, 400)
# Navigate to the url
driver.get('/tmp/selenium/index-16.html')
# Take a screenshot
driver.save_screenshot("screenshot-1.png")
# Get the radio button whose value="Chemistry"
radio_button = driver.find_element(By.XPATH, "//input[@type='radio' and @value='Chemistry']")
# Click the radio button
radio_button.click()
# Take a screenshot
driver.save_screenshot("screenshot-2.png")
# Close the driver
driver.quit()
screenshot-1.png - Before selecting the radio button
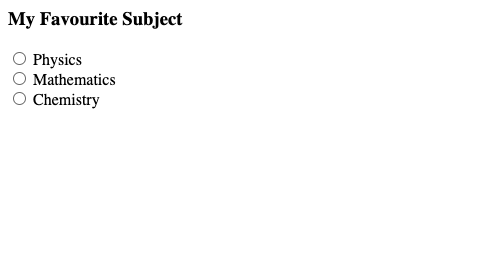
screenshot-2.png - After selecting the radio button
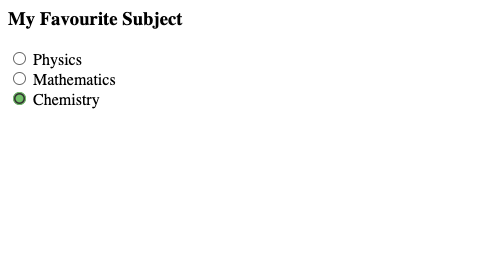
Summary
In this Python Selenium tutorial, we have given instructions on how to select a radio button by value attribute, with an example.