Pressing Browser's Back Button - Selenium
Pressing the Browser's Back Button
To simulate pressing of the browser's back button programmatically using Selenium for Python, call back()
method on the Web Driver object.
driver.back()
Example
- Initialise Chrome Web Driver object as
driver
. - Get the URL
/
using webdriver
object. - Find element by link text
'OpenCV'
and click on the element. - Make an implicit wait of 5 seconds.
- Press browser's back button using
driver.back()
.
Python Program
from selenium.webdriver.chrome.service import Service
from selenium import webdriver
from selenium.webdriver.common.by import By
service = Service(executable_path="/usr/local/bin/chromedriver")
#initialize web driver
with webdriver.Chrome(service=service) as driver:
#navigate to the url
driver.get('/')
#find element that has link text : 'OpenCV'
driver.find_element(By.LINK_TEXT, 'OpenCV').click()
#press on browser's back button
driver.back()
Screenshots
Navigation Step #1 : Get the URL /
.
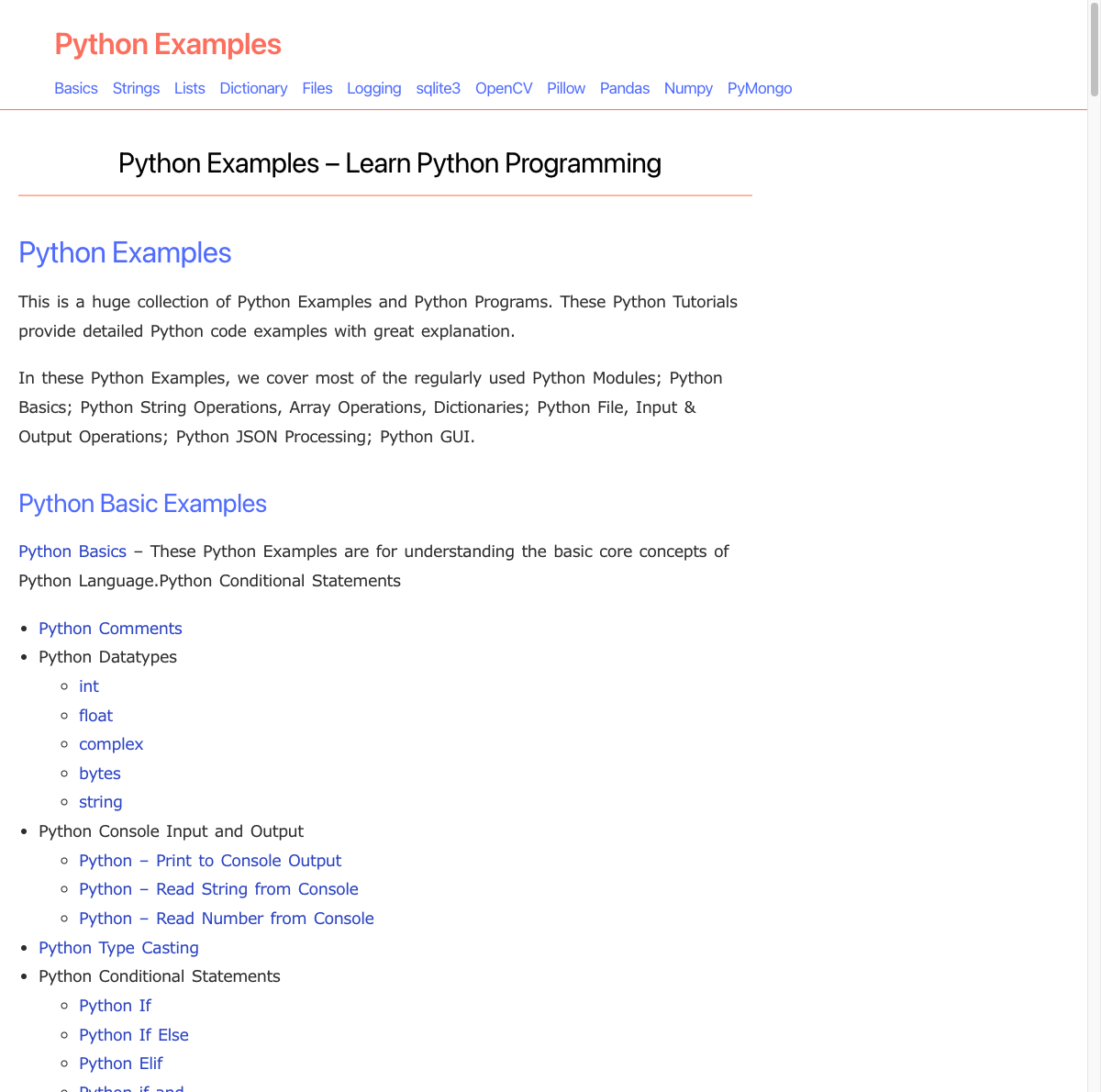
Navigation Step #2 : Find OpenCV link and click on it.
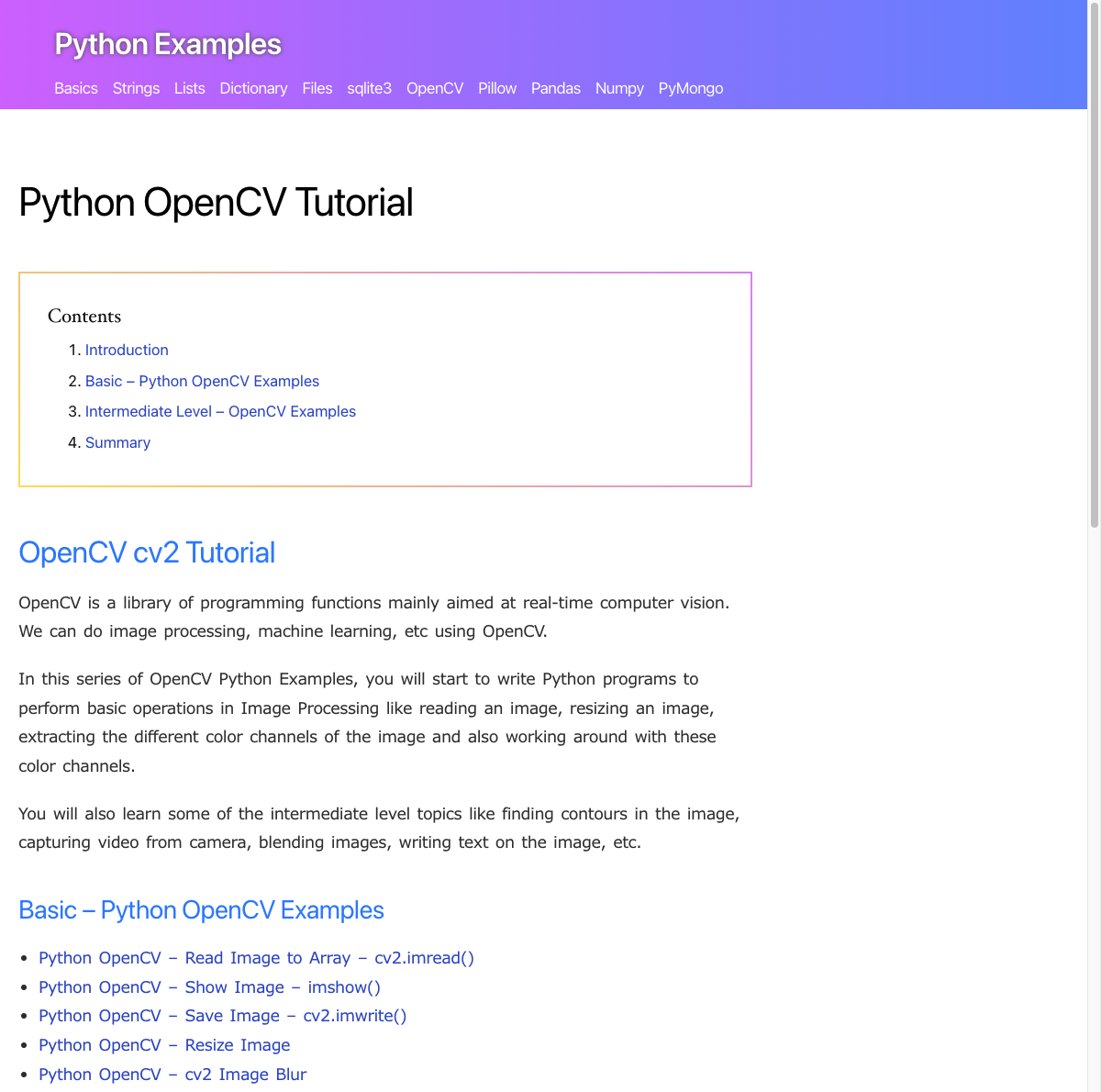
Navigation Step #3 : Press the browser's back button.
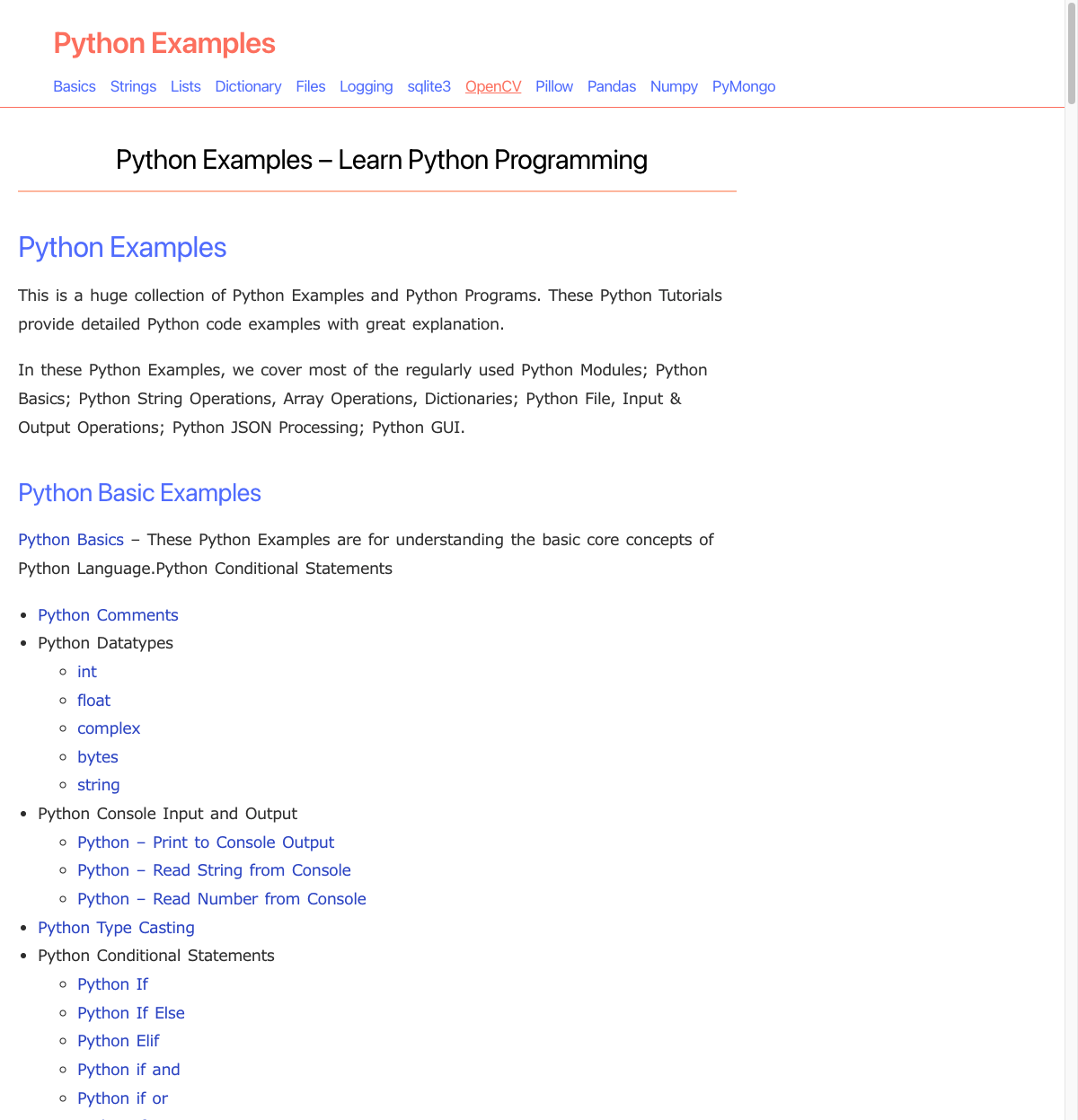
Summary
In this Python Selenium Tutorial, we learned how to press the browser's back button programmatically using driver.back()
method.