Get Current URL using Selenium
Get Current URL
To get the current URL in the browser window using Selenium for Python, read the current_url property of web driver object.
driver.current_url
Example
- Initialise Chrome Web Driver object as
driver
. - Get the URL
/
using webdriver
object. - Find element by link text
'OpenCV'
and click on the element. - Make an implicit wait of 5 seconds.
- Get the current URL to
my_current_url
variable. - Print the current URL to output.
Python Program
from selenium.webdriver.chrome.service import Service
from selenium import webdriver
from selenium.webdriver.common.by import By
service = Service(executable_path="/usr/local/bin/chromedriver")
with webdriver.Chrome(service=service) as driver:
driver.get('/')
driver.find_element(By.LINK_TEXT, 'OpenCV').click()
driver.implicitly_wait(5)
my_current_url = driver.current_url
print(my_current_url)
Output
https://pythonexamples.org/python-opencv/
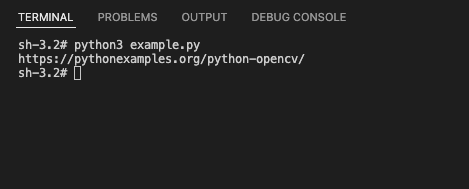
Summary
In this Python Selenium Tutorial, we learned how to navigate to a specific URL using driver.get()
method.