Image.show() Show or Display Image - Python Pillow Examples
Python - Display Image using PIL
In Python, to display an image using the Pillow (PIL) library, the show()
method is used. This method opens the default image viewer application on your computer to display the image. The image is first written to a temporary file, and once the program execution is completed, the temporary file is automatically deleted.
Examples
1. Show or display a given image
In this example, we will read an image and display it using the show()
method.
Python Program
from PIL import Image
# Read the image
im = Image.open("sample-image.png")
# Show image
im.show()
Explanation:
- The image is opened using
Image.open()
. - The
show()
method is then called on the image object to open the default program for displaying images on the system. - The temporary file containing the image is opened in the system's default image viewer program, such as Photos on Windows or Preview on macOS.
Output
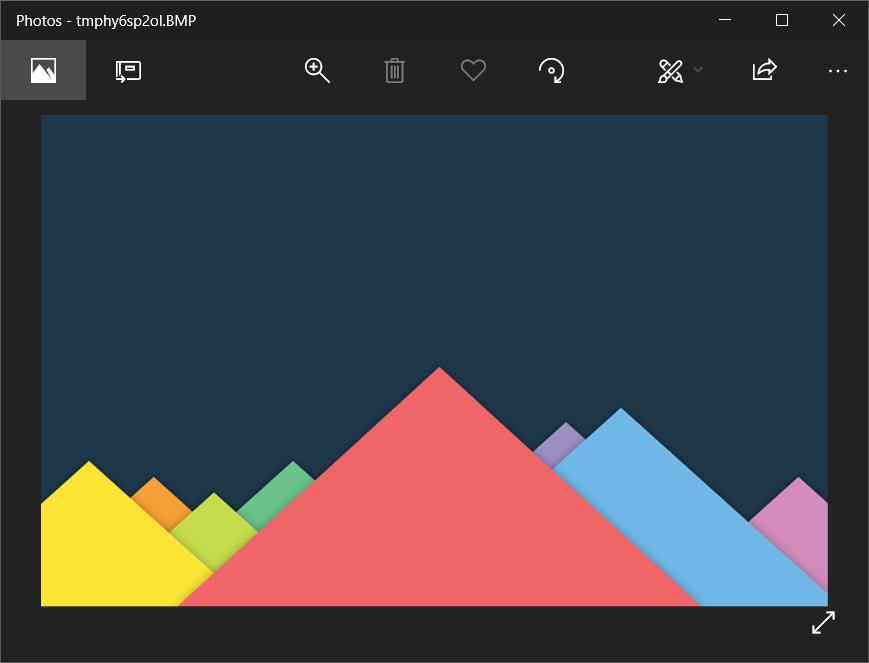
In this example, the system's default program (e.g., Photos on Windows) is used to display the image.
2. Display multiple images using show()
You can also display more than one image at a time. Each image will open in its own instance of the default image viewer program, stacked up on the screen.
In the following example, we read and display two images simultaneously.
Python Program
from PIL import Image
# Read multiple images
im1 = Image.open("sample-image.png")
im2 = Image.open("test-image.png")
# Show the images
im1.show()
im2.show()
Explanation:
- The two images are read using
Image.open()
and stored in separate variables,im1
andim2
. - The
show()
method is called on each image object, which will open each image in its own viewer window. - Both images will be displayed simultaneously, each in a separate instance of the default image viewer program.
3. Display an image after processing
In this example, we apply some image processing (e.g., rotating) before displaying the image using the show()
method. This demonstrates the ability to display an image after modifying it.
Python Program
from PIL import Image
# Read the image
im = Image.open("sample-image.png")
# Apply a transformation (e.g., rotate)
im_rotated = im.rotate(90)
# Show the rotated image
im_rotated.show()
Explanation:
- The image is opened using
Image.open()
. - A 90-degree rotation is applied using the
rotate()
method. - The rotated image is displayed using
show()
method, which will open the modified image in the default viewer program.
Summary
In this tutorial, we learned how to display images using the show()
method from the Pillow library. We explored different use cases, such as displaying a single image, multiple images, and images after applying transformations.