How to blur an image in Pillow?
Pillow - Blur image
To blur an image using Pillow library, you can use Image.filter() function with ImageFilter.BLUR kernel filter.
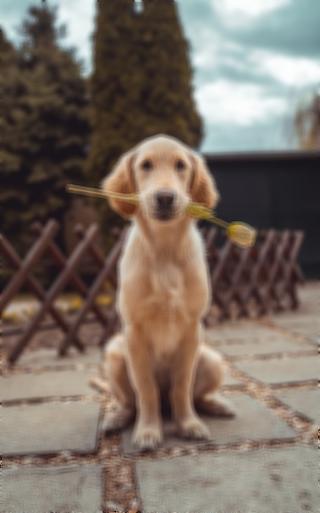
Steps to blur an image
Follow these steps to blur a given image.
- Import Image, and ImageFilter modules from Pillow library.
- Read input image using
Image.open()
function. - Call
filter()
function on the read Image object and passImageFilter.BLUR
as argument to the function. The function returns blurred image as PIL.Image.Image object. - Save the returned image to required location using
Image.save()
function.
Syntax of filter() function
The syntax of filter() function from PIL.Image module is
PIL.Image.filter(filter_kernel)
Parameter | Description |
---|---|
filter_kernel | A filter kernel that applies to the given image. To blur the image, you can use ImageFilter.BLUR filter kernel. |
Returns
The function returns a PIL.Image.Image object.
Examples
1. Blur a given image
In the following example, we read an image test_image.jpg
, blur this image using Image.filter() function, and save the blurred image as blurred_image.jpg
.
Python Program
from PIL import Image, ImageFilter
# Open the image
image = Image.open("test_image.jpg")
# Blur the image
blurred_image = image.filter(ImageFilter.BLUR)
# Save the resulting image
blurred_image.save("blurred_image.jpg")
Original Image [test_image.jpg]
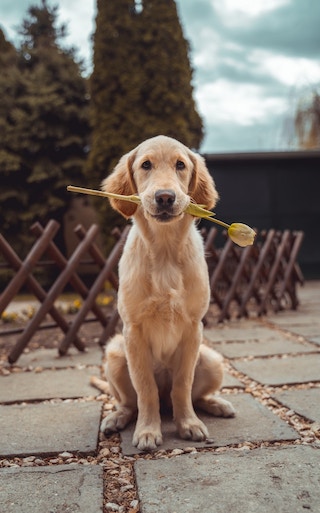
Resulting Image with BLUR effect [blurred_image.jpg]
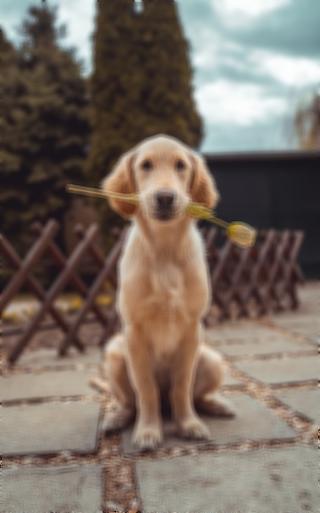
Summary
In this Python Pillow Tutorial, we learned how to blur a given image using PIL.Image.filter() function, with the help of examples.