Adjust Image Sharpness - Pillow - Python Examples
Python Pillow - Sharpen Image
You can change the sharpness of an image using the ImageEnhance
class from the PIL library. This tutorial will guide you on how to sharpen or blur an image with the help of examples.
Steps to Sharpen Image using PIL
To adjust image sharpness using Python Pillow, follow these steps:
- Read the image using
Image.open()
. - Create an
ImageEnhance.Sharpness()
enhancer for the image. - Enhance the image sharpness using the
enhance()
method, by setting the required factor.
The factor determines the level of sharpness: a factor greater than 1 sharpens the image, while a factor less than 1 blurs it. A factor of 1 preserves the original image's sharpness.
Examples
1. Increase sharpness of the given image
In this example, we will increase the sharpness of the given image by a factor of 2, which will result in a sharpened image.
Python Program
from PIL import Image, ImageEnhance
im = Image.open("original-image.png")
enhancer = ImageEnhance.Sharpness(im)
factor = 1
im_s_1 = enhancer.enhance(factor)
im_s_1.save('original-image-1.png');
factor = 2
im_s_1 = enhancer.enhance(factor)
im_s_1.save('sharpened-image.png');
Explanation:
- First, we open the image file using
Image.open()
. - We create an
ImageEnhance.Sharpness()
object to enhance the image sharpness. - We then call
enhance()
with a factor of 2, which sharpens the image. - Finally, the sharpened image is saved with a new name,
sharpened-image.png
.
Original Image - original-image.png
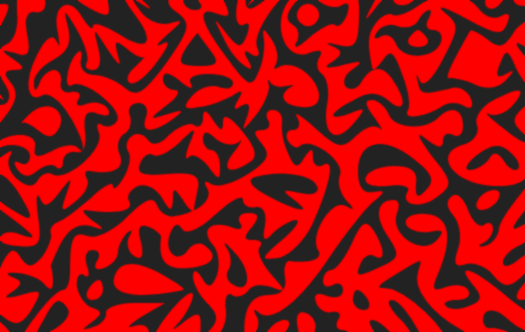
Sharpened Image - sharpened-image.png
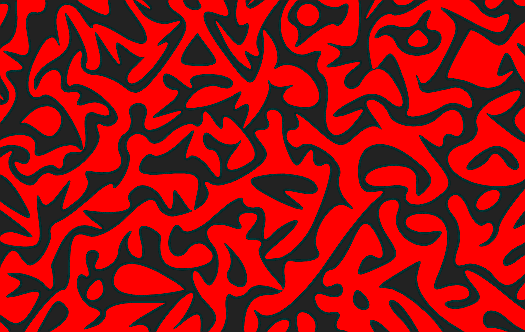
2. Decrease sharpness of the given image
In this example, we will decrease the sharpness of the given image by a factor of 0.05, resulting in a blurred image.
Python Program
from PIL import Image, ImageEnhance
im = Image.open("original-image.png")
enhancer = ImageEnhance.Sharpness(im)
factor = 1
im_s_1 = enhancer.enhance(factor)
im_s_1.save('original-image-1.png');
factor = 0.05
im_s_1 = enhancer.enhance(factor)
im_s_1.save('blurred-image.png');
Explanation:
- Again, the image is opened using
Image.open()
. - An
ImageEnhance.Sharpness()
enhancer is created. - We first apply the original sharpness by setting the factor to 1.
- Next, we apply a factor of 0.05, which reduces the sharpness, resulting in a blurred image.
- The blurred image is saved with the name
blurred-image.png
.
Original Image - original-image.png
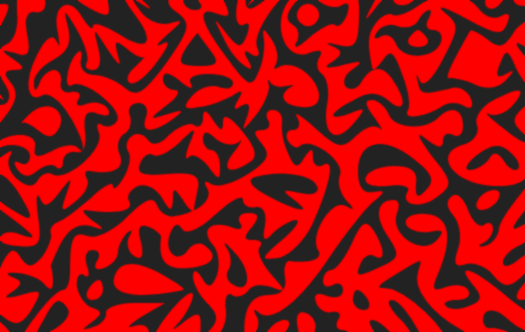
Blurred Image - blurred-image.png
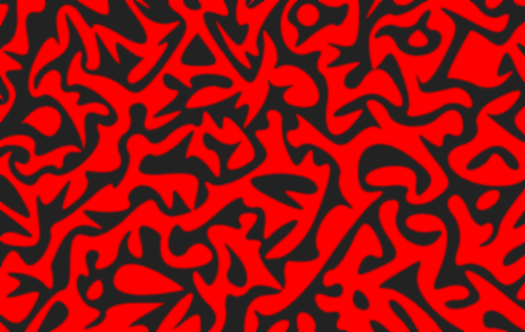
3. Applying a moderate sharpness adjustment
In this example, we apply a moderate level of sharpness enhancement to see the difference more clearly.
Python Program
from PIL import Image, ImageEnhance
im = Image.open("original-image.png")
enhancer = ImageEnhance.Sharpness(im)
factor = 1.5
im_s_1 = enhancer.enhance(factor)
im_s_1.save('moderate-sharpness-image.png');
Explanation:
- This time, we use a factor of 1.5, which enhances the sharpness moderately.
- The resulting image is saved as
moderate-sharpness-image.png
.
Summary
In this tutorial, we learned how to adjust the sharpness of an image using the ImageEnhance.Sharpness()
function in the Python Pillow library. By adjusting the enhancement factor, we can sharpen or blur the image based on our requirements.