Change Image Contrast using Pillow - Python Examples
Pillow - Change Image Contrast
Contrast refers to the difference between the darkest and lightest parts of an image. By adjusting contrast, you make the colors more vivid or more subdued. Increasing contrast makes the bright areas brighter and dark areas darker, while decreasing contrast makes the image appear greyer.
In this tutorial, we will learn how to change the contrast of an image using the ImageEnhance
class from the Pillow library.
Steps to Adjust Image Contrast
To adjust image contrast using Python Pillow, follow these steps:
- Read the image using
Image.open()
. - Create an
ImageEnhance.Contrast()
enhancer for the image. - Enhance the image contrast using the
enhance()
method, specifying the required factor.
By adjusting the factor, you can control the contrast of the image. A factor of 1 preserves the original contrast. Factors less than 1 reduce the contrast, while factors greater than 1 increase the contrast.
Examples
1. Increase the contrast of the given image
In this example, we will increase the contrast of the image by a factor of 1.5, which results in a more vivid contrast.
Python Program
from PIL import Image, ImageEnhance
# Read the image
im = Image.open("sample-image.png")
# Image contrast enhancer
enhancer = ImageEnhance.Contrast(im)
factor = 1 # Original contrast
im_output = enhancer.enhance(factor)
im_output.save('original-image.png')
factor = 1.5 # Increase contrast
im_output = enhancer.enhance(factor)
im_output.save('more-contrast-image.png')
Explanation:
- The image is opened using
Image.open()
. - An
ImageEnhance.Contrast()
enhancer is created to adjust the contrast. - The original contrast is preserved with a factor of 1.
- The contrast is increased by setting the factor to 1.5, making the bright areas brighter and dark areas darker.
- The enhanced image is saved as
more-contrast-image.png
.
Original Image
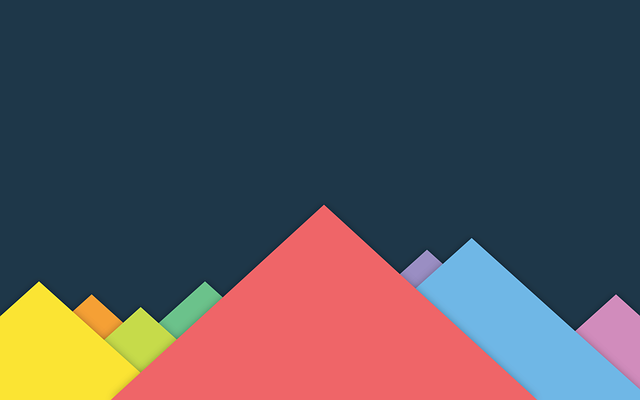
Image with Increased Contrast
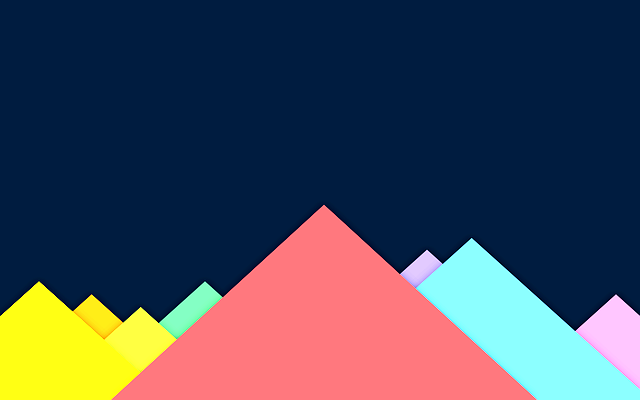
2. Decrease the contrast of the given image
In this example, we decrease the contrast of the image by a factor of 0.5, which results in a more subdued, greyed-out appearance.
Python Program
from PIL import Image, ImageEnhance
# Read the image
im = Image.open("sample-image.png")
# Image contrast enhancer
enhancer = ImageEnhance.Contrast(im)
factor = 1 # Original contrast
im_output = enhancer.enhance(factor)
im_output.save('original-image.png')
factor = 0.5 # Decrease contrast
im_output = enhancer.enhance(factor)
im_output.save('less-contrast-image.png')
Explanation:
- The image is opened using
Image.open()
. - An
ImageEnhance.Contrast()
enhancer is created for contrast adjustment. - The contrast is initially preserved with a factor of 1.
- The contrast is decreased by setting the factor to 0.5, making the image appear greyer and duller.
- The darkened, low-contrast image is saved as
less-contrast-image.png
.
Original Image
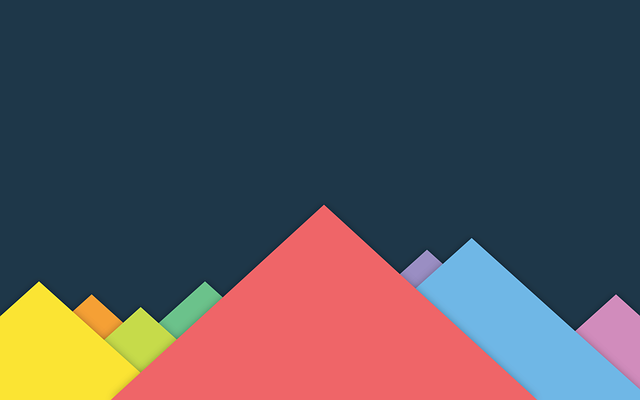
Dull Image - With decreased contrast
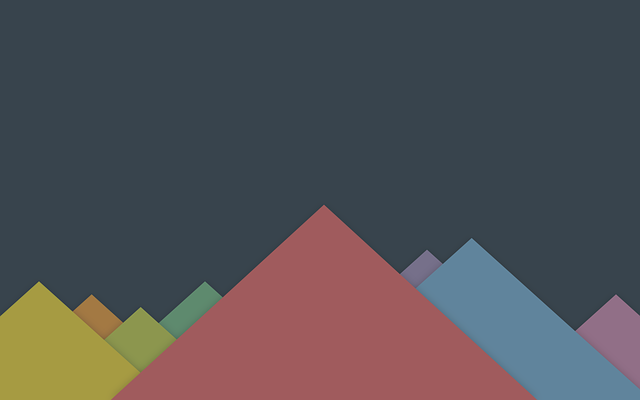
3. Apply slight contrast increase
In this example, we apply a slight contrast increase by using a factor of 1.2.
Python Program
from PIL import Image, ImageEnhance
# Read the image
im = Image.open("sample-image.png")
# Image contrast enhancer
enhancer = ImageEnhance.Contrast(im)
factor = 1 # Original contrast
im_output = enhancer.enhance(factor)
im_output.save('original-image.png')
factor = 1.2 # Slightly increase contrast
im_output = enhancer.enhance(factor)
im_output.save('slightly-contrast-image.png')
Explanation:
- The image is opened and an
ImageEnhance.Contrast()
enhancer is created. - The original contrast is retained with a factor of 1.
- A slight contrast increase is applied by setting the factor to 1.2, making the image's colors more vivid but not too extreme.
- The result is saved as
slightly-contrast-image.png
.
Summary
In this tutorial, we explored how to adjust the contrast of an image using the ImageEnhance.Contrast()
method from the Pillow library. We covered examples of increasing and decreasing the contrast, as well as applying a slight contrast adjustment.