Adjust Image Brightness using Pillow - Python Examples
Python - Adjust Image Brightness using Pillow Library
You can adjust the brightness of an image using the Python Pillow library. Adjusting the brightness involves either increasing or decreasing the pixel values evenly across all channels of the entire image. Increasing the brightness makes the image appear whiter, while decreasing it makes the image darker.
Steps to Adjust Image Brightness using PIL
To adjust image brightness using Python Pillow, follow these steps:
- Read the image using
Image.open()
. - Create an
ImageEnhance.Brightness()
enhancer for the image. - Enhance the image brightness using the
enhance()
method, by setting the required factor.
By adjusting the factor, you can brighten or darken the image. A factor of 1 retains the original brightness, while a factor greater than 1 brightens the image, and a factor less than 1 darkens the image.
Examples
1. Increase the brightness of the given image
In the following example, we will increase the brightness of the image by a factor of 1.5, resulting in a brightened image.
Python Program
from PIL import Image, ImageEnhance
# Read the image
im = Image.open("sample-image.png")
# Image brightness enhancer
enhancer = ImageEnhance.Brightness(im)
factor = 1 # Original brightness
im_output = enhancer.enhance(factor)
im_output.save('original-image.png')
factor = 1.5 # Increase brightness
im_output = enhancer.enhance(factor)
im_output.save('brightened-image.png')
Explanation:
- First, the image is opened using
Image.open()
. - An
ImageEnhance.Brightness()
enhancer is created to adjust the brightness. - The brightness is first preserved with a factor of 1.
- Then, the brightness is increased by setting the factor to 1.5, which brightens the image.
- Finally, the brightened image is saved with the name
brightened-image.png
.
Original Image
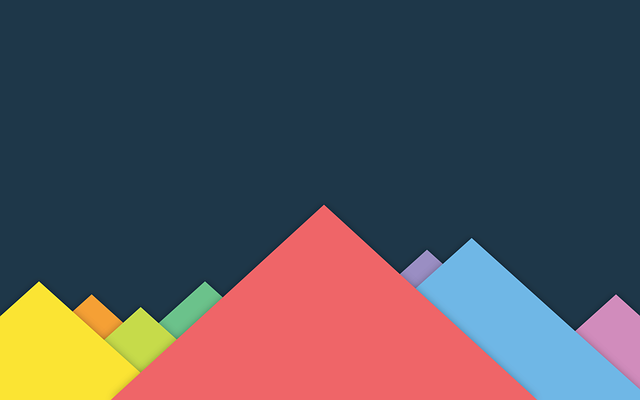
Brightened Image - With increased brightness
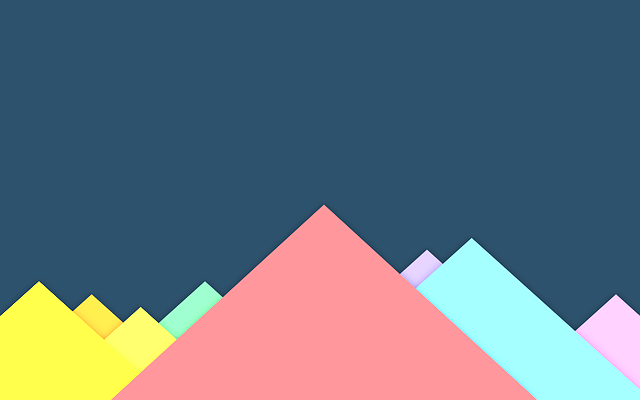
2. Decrease the brightness of the given image
In the following example, we will decrease the brightness of the image by a factor of 0.5, resulting in a darker image.
Python Program
from PIL import Image, ImageEnhance
# Read the image
im = Image.open("sample-image.png")
# Image brightness enhancer
enhancer = ImageEnhance.Brightness(im)
factor = 1 # Original brightness
im_output = enhancer.enhance(factor)
im_output.save('original-image.png')
factor = 0.5 # Decrease brightness
im_output = enhancer.enhance(factor)
im_output.save('darkened-image.png')
Explanation:
- The image is opened using
Image.open()
. - An
ImageEnhance.Brightness()
enhancer is created to adjust the image's brightness. - First, the original brightness is retained by setting the factor to 1.
- Then, the brightness is decreased by setting the factor to 0.5, which darkens the image.
- The darkened image is saved with the name
darkened-image.png
.
Original Image
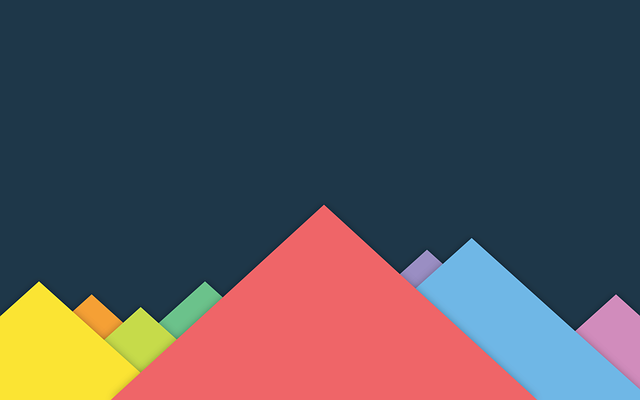
Darkened Image - With decreased brightness
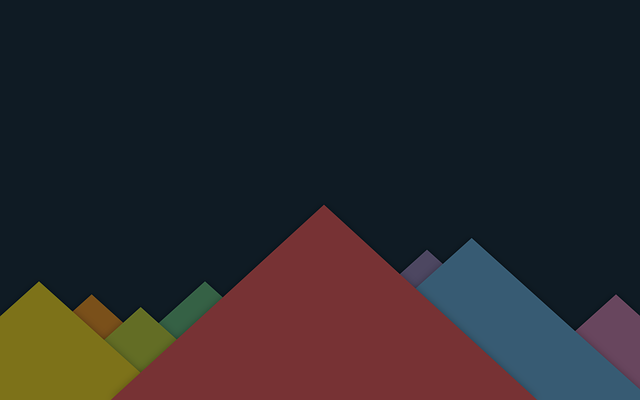
3. Apply a slight brightness increase
In this example, we apply a moderate brightness increase by using a factor of 1.2.
Python Program
from PIL import Image, ImageEnhance
# Read the image
im = Image.open("sample-image.png")
# Image brightness enhancer
enhancer = ImageEnhance.Brightness(im)
factor = 1 # Original brightness
im_output = enhancer.enhance(factor)
im_output.save('original-image.png')
factor = 1.2 # Slightly increase brightness
im_output = enhancer.enhance(factor)
im_output.save('slightly-brightened-image.png')
Explanation:
- As before, the image is opened and an
ImageEnhance.Brightness()
enhancer is created. - We first preserve the original brightness with a factor of 1.
- Then, we apply a slight brightness increase with a factor of 1.2, which makes the image slightly brighter.
- The result is saved as
slightly-brightened-image.png
.
Summary
In this tutorial, we learned how to adjust the brightness of an image using the ImageEnhance.Brightness()
function in the Python Pillow library. By adjusting the enhancement factor, we can brighten or darken the image based on the required outcome.