Select only specific columns from table in Python MySQL
Python MySQL - Select only specific columns from table
To select only specific columns from a MySQL table in Python program,
- Create a connection to the MySQL database with user credentials and database name, using connect() function.
- Get cursor object to the database using cursor() function.
- Call execute() function on the cursor object, and pass the SELECT FROM table query. This SELECT query specifies the columns that we need to fetch from the table.
- Call fetchall() function on the cursor object. The function returns an iterator to the records in the table, where each record is a tuple.
Example
Consider that there is a schema named mydatabase
in MySQL. The credentials to access this database are, user: root
and password: admin1234
, and there is a table named fruits
in mydatabase
.
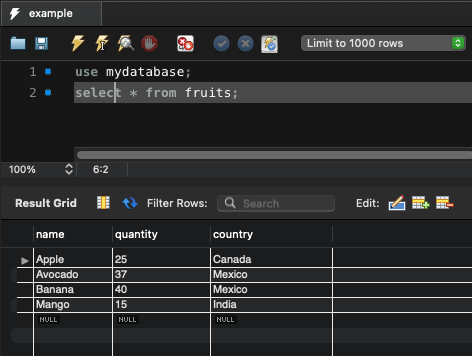
In the following program, we fetch only name
and quantity
columns from the table.
Python Program
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="admin1234",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT name, quantity FROM fruits")
myresult = mycursor.fetchall()
for row in myresult:
print(row)
Output
('Apple', 25)
('Avocado', 37)
('Banana', 40)
('Mango', 15)
Summary
In this tutorial of Python Examples, we learned how to select only specific columns from a table in MySQL database, from a Python program.