Get Rows from Table based on Condition in Python MySQL
Python MySQL - Get rows from Table based on condition
To get rows from MySQL table based on a condition in Python,
- Create a connection to the MySQL database with user credentials and database name, using connect() function.
- Get cursor object to the database using cursor() function.
- Call execute() function on the cursor object, and pass the SELECT FROM table query with WHERE clause.
- Call fetchall() function on the cursor object. The function returns an iterator to the records in the table. We can use a For loop on this iterator object.
Example
Consider that there is a schema named mydatabase
in MySQL. The credentials to access this database are, user: root
and password: admin1234
, and there is a table named fruits
in mydatabase
.
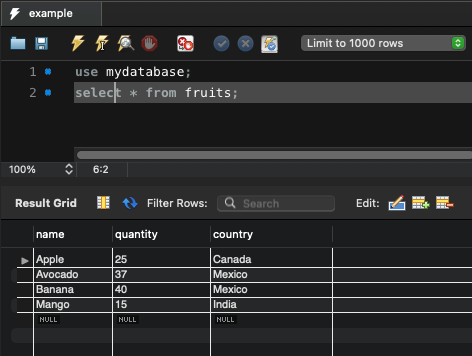
In this example, we get the rows from fruits
table where country
is Mexico
.
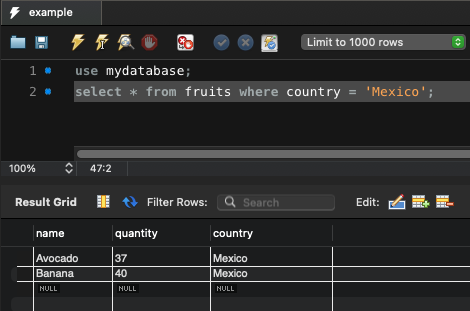
Python Program
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="admin1234",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM fruits WHERE country='Mexico'")
myresult = mycursor.fetchall()
for row in myresult:
print(row)
Output
('Avocado', 37, 'Mexico')
('Banana', 40, 'Mexico')
Summary
In this tutorial of Python Examples, we learned how to get the records from a table based on a condition in MySQL database, from a Python program.