Drop Table in Python MySQL
Python MySQL - Drop table
To delete/drop a table in MySQL from a Python program,
- Create a connection to the MySQL database with user credentials and database name, using connect() function.
- Get cursor object to the database using cursor() function.
- Call execute() function on the cursor object, and pass the DROP table query with the old table name as argument. The DROP table query deletes the table from the database.
Example
Consider that there is a schema named mydatabase
in MySQL. The credentials to access this database are, user: root
and password: admin1234
, and there is a table named fruits
in mydatabase
.
In the following program, we drop the fruits
table from the database.
Python Program
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="admin1234",
database="mydatabase"
)
mycursor = mydb.cursor()
try:
mycursor.execute("DROP TABLE fruits")
print('Table dropped successfully.')
except:
print('An exception occurred while dropping table.')
mydb.commit()
Output
Table dropped successfully.
Database before Dropping Table
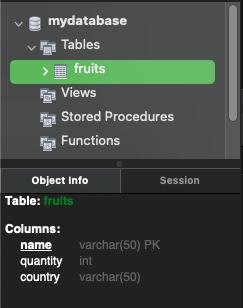
Database after Dropping Table
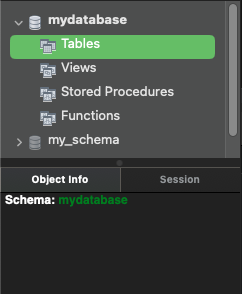
Summary
In this tutorial of Python Examples, we learned how to drop a table from database in MySQL, from a Python program.