Python Matplotlib - Bar Plot Display Values above Bars
Python Matplotlib - Bar Plot Display Values above Bars
Displaying values above bars in a bar plot is a common requirement for making data visualizations more informative and easier to interpret. Python's Matplotlib library allows you to achieve this with ease. This tutorial demonstrates how to add values above bars and customize their appearance.
Adding Values Above Bars
To display values above bars, use the plt.text()
function or iterate through the bars and position the text appropriately.
Example 1: Display Basic Values Above Bars
import matplotlib.pyplot as plt
# Data for the bar plot
categories = ['Category A', 'Category B', 'Category C', 'Category D']
values = [10, 20, 15, 25]
# Create the bar plot
bars = plt.bar(categories, values, color='skyblue', edgecolor='black')
# Add values above bars
for bar in bars:
yval = bar.get_height()
plt.text(bar.get_x() + bar.get_width() / 2, yval + 0.5, str(yval), ha='center', va='bottom')
# Add labels and title
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot with Values Above Bars')
# Show the plot
plt.show()
Explanation
bar.get_height()
retrieves the height of each bar.plt.text()
places the value above the bar using its x and y coordinates.- Horizontal and vertical alignment is set using
ha
andva
to center the text.
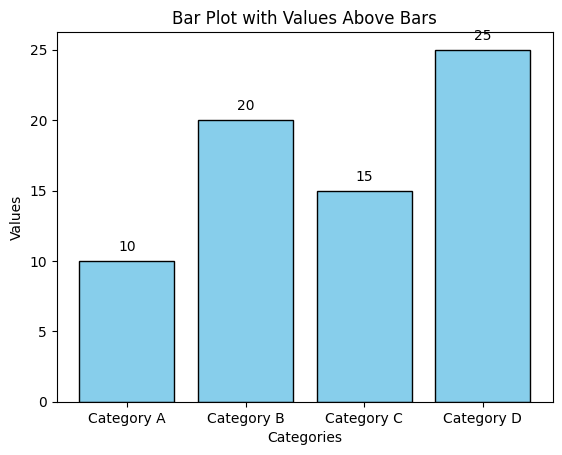
Customizing the Display
You can customize the text appearance, such as font size, color, and style, for better readability and aesthetics.
Example 2: Customized Text Appearance
import matplotlib.pyplot as plt
# Data for the bar plot
categories = ['Category A', 'Category B', 'Category C', 'Category D']
values = [10, 20, 15, 25]
# Create the bar plot
bars = plt.bar(categories, values, color='orange', edgecolor='black')
# Add values above bars with customization
for bar in bars:
yval = bar.get_height()
plt.text(bar.get_x() + bar.get_width() / 2, yval + 0.5, f"{yval} units",
ha='center', va='bottom', fontsize=10, color='red', fontweight='bold')
# Add labels and title
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot with Customized Values Above Bars')
# Show the plot
plt.show()
Explanation
- Text is formatted using an f-string to add a unit label (e.g.,
"units"
). - Font size, color, and weight are customized using
fontsize
,color
, andfontweight
.
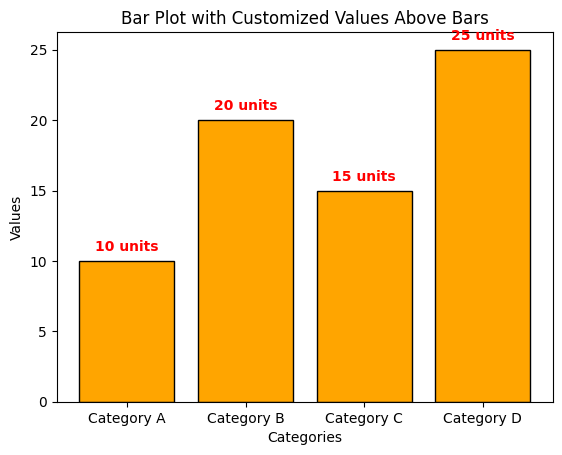
Handling Narrow Bars
For narrow bars, placing values above them requires careful positioning to ensure visibility.
Example 3: Adjusting for Narrow Bars
import matplotlib.pyplot as plt
# Data for the bar plot
categories = ['Category A', 'Category B', 'Category C', 'Category D']
values = [100, 200, 150, 250]
bar_width = 0.3
# Create the bar plot
bars = plt.bar(categories, values, width=bar_width, color='green', edgecolor='black')
# Add values above narrow bars
for bar in bars:
yval = bar.get_height()
plt.text(bar.get_x() + bar.get_width() / 2, yval + 10, f"{yval}",
ha='center', va='bottom', fontsize=8, color='blue')
# Add labels and title
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot with Adjusted Values for Narrow Bars')
# Show the plot
plt.show()
Explanation
- The bar width is reduced using the
width
parameter. - The text position is adjusted by increasing the y-offset to prevent overlap with the bar.
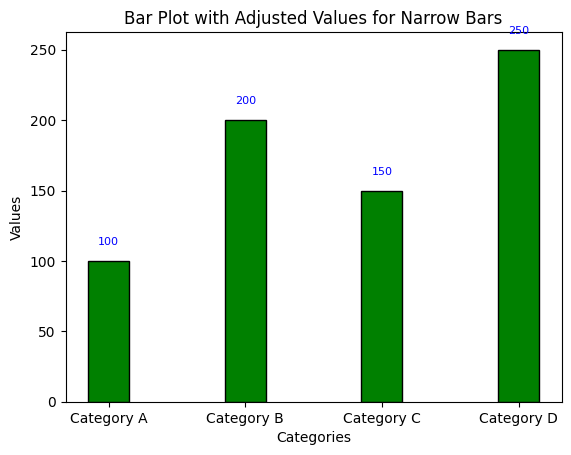
Summary
In this tutorial, we covered:
- How to display values above bars in a bar plot.
- Customizing text appearance to enhance readability.
- Handling narrow bars by adjusting the text position.
Adding values above bars can make your bar plots more informative and engaging.