Python Matplotlib - Adding Legend to Bar Plot
Python Matplotlib - Adding Legend to Bar Plot
Adding legends to bar plots helps to improve the clarity of the plot, especially when you are plotting multiple datasets. In Matplotlib, you can easily add a legend to bar plots to identify the different categories or groups in the plot.
In this tutorial, we will learn:
- How to add a legend to a bar plot.
- How to customize the legend appearance and position.
Adding a Simple Legend to a Bar Plot
The legend()
function is used in Matplotlib to add a legend. By default, it places the legend in the best location based on the plot's content.
Example 1: Basic Bar Plot with a Legend
import matplotlib.pyplot as plt
# Data for the bar plot
categories = ['Python', 'Java', 'C++', 'Ruby']
values1 = [40, 30, 20, 10]
values2 = [30, 40, 25, 5]
# Create bar plot for first dataset
bars1 = plt.bar(categories, values1, label='2024 Data')
# Create bar plot for second dataset
bars2 = plt.bar(categories, values2, bottom=values1, label='2023 Data')
# Add labels and title
plt.xlabel('Programming Languages')
plt.ylabel('Popularity (%)')
plt.title('Popularity of Programming Languages Over Two Years')
# Add a legend
plt.legend()
# Show the plot
plt.show()
Explanation
- The
label
parameter is added to eachbar()
function to specify the labels for the legend. - The
legend()
function is called to display the legend on the plot. - By default, the legend is placed at the best position on the plot.
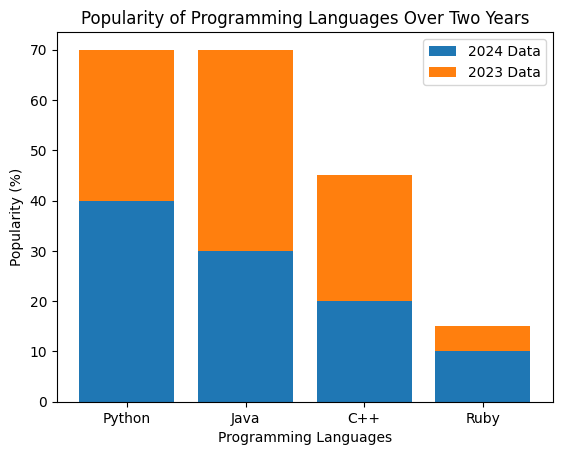
Customizing the Legend Position
You can change the position of the legend on the plot using the loc
parameter in the legend()
function. The loc
parameter accepts several predefined location strings.
Example 2: Customizing Legend Position
import matplotlib.pyplot as plt
# Data for the bar plot
categories = ['Python', 'Java', 'C++', 'Ruby']
values1 = [40, 30, 20, 10]
values2 = [30, 40, 25, 5]
# Create bar plot for first dataset
plt.bar(categories, values1, label='2024 Data')
# Create bar plot for second dataset
plt.bar(categories, values2, bottom=values1, label='2023 Data')
# Add labels and title
plt.xlabel('Programming Languages')
plt.ylabel('Popularity (%)')
plt.title('Popularity of Programming Languages Over Two Years')
# Add a legend at the top-left corner
plt.legend(loc='upper left')
# Show the plot
plt.show()
Explanation
- The
loc='upper left'
argument places the legend at the top-left corner of the plot. - Other options for
loc
include 'upper right', 'lower left', 'lower right', etc.
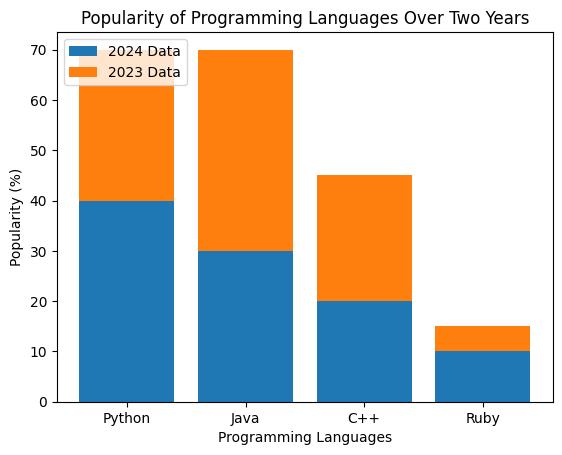
Summary
In this tutorial, we covered:
- How to add a basic legend to a bar plot using the
legend()
function. - Customizing the position of the legend using the
loc
parameter.
Adding legends to bar plots helps improve readability and makes it easier to interpret the data, especially when working with multiple datasets or categories.