Redirect URL in Python Flask
Flask - Redirect URL
In a flask application, we can redirect a URL to another URL using redirect()
function.
The following is a simple code snippet to redirect /page-1
to /page-2
.
@app.route('/page-1')
def page2():
return redirect("/page-2", code=302)
The second argument to redirect function specifies the type of redirect (temporary or permanent). Redirect code 301
indicates that page has been permanently moved to a new location, whereas redirect code 302
indicates that the page is moved to a new location temporarily.
We must import redirect from flask library before using it.
Example
In this example, we build a flask application where we have following web pages.
- page-1.html
- page-2.html
When a request comes for page-1.html
, we will redirect it to page-2.html
, using redirect()
function.
Project Structure
The following is the structure of our flask application.
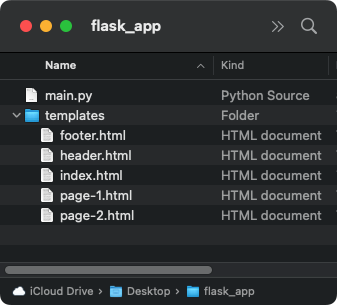
main.py
from flask import Flask, render_template, redirect
app = Flask(__name__)
@app.route("/page-1")
def page_1():
return redirect("/page-2", code=302)
@app.route("/page-2")
def page_2():
return render_template("page-2.html")
if __name__ == "__main__":
app.run(host="127.0.0.1", port=8080, debug=True)
page-1.html
{% include "header.html" %}
<h1>Page 1</h1>
<h2>About</h2>
<p>Welcome to sample flask application.</p>
{% include "footer.html" %}
page-2.html
{% include "header.html" %}
<h1>Page 2</h1>
<h2>Another Page</h2>
<p>This is another page in this flask application.</p>
{% include "footer.html" %}
Terminal
Open terminal at the root of the application, and run the Python file main.py.
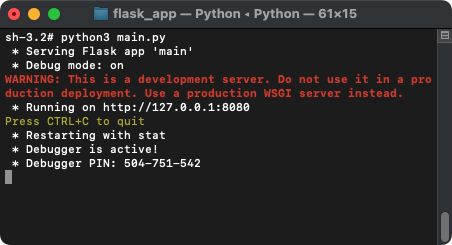
Now, open a browser of your choice and hit the URL http://127.0.0.1:8080/page-1
.
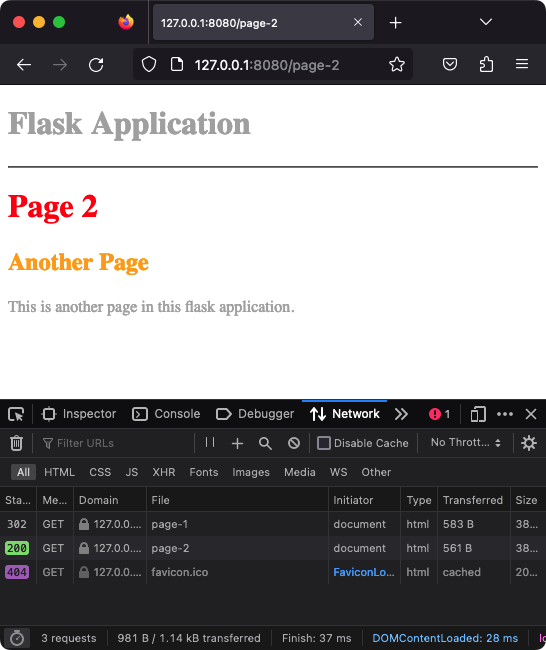
As you can observe under the Network tab in browser, the server responds to the request URL /page-1
with a 302
redirect, just like what we said it to do, and redirects it to /page-2
.
Project ZIP
Summary
In this Python Flask Tutorial, we learned how to redirect a URL, with the help of an example flask application.