How to copy a File and Rename?
Python - Copy File
To copy a file from one location to another in Python, import shutil library, call shutil.copy(source, target)
function.
shutil.copy() copies the source
file to the file path specified by target
. The syntax to use shutil.copy() function is
import shutil
shutil.copy(source, target)
where source
and target
are absolute, or relative file paths.
To preserve the meta information of the original file, we can use shutil.copy2() function. shutil.copy2() copies the source
file to the file path specified by target
.
shutil.copy2(source, target)
Examples
1. Copy File
In the following example, we will copy the file at location myfolder/sample.txt
to myfolder/sample_2.txt
.
Original File
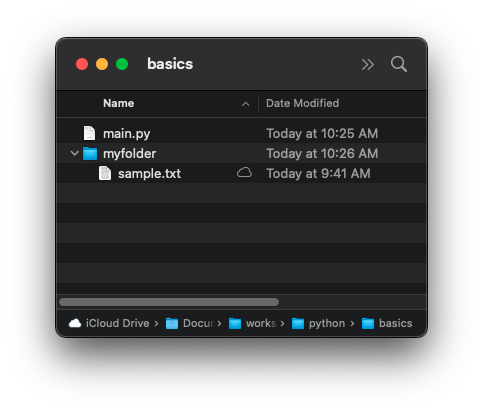
Python Program
import shutil
source = 'myfolder/sample.txt'
target = 'myfolder/sample_2.txt'
try :
shutil.copy(source, target)
print('File copied successfully.')
except:
print('Could not copy file.')
print('An exception occurred.')
Output
File copied successfully.
Copied File
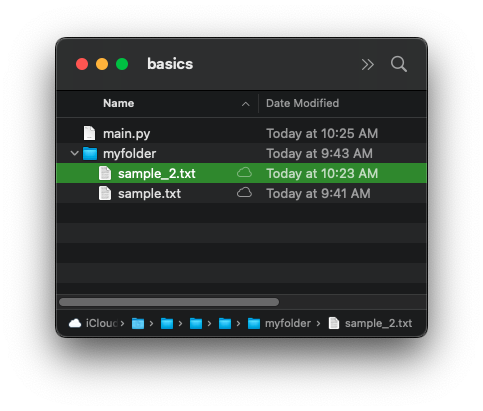
2. Copy File with original Meta preserved
In the following example, we will copy the file at location a/b/sample.txt
to c/d/sample.txt
with the meta of the copied file same as the original file.
Original File
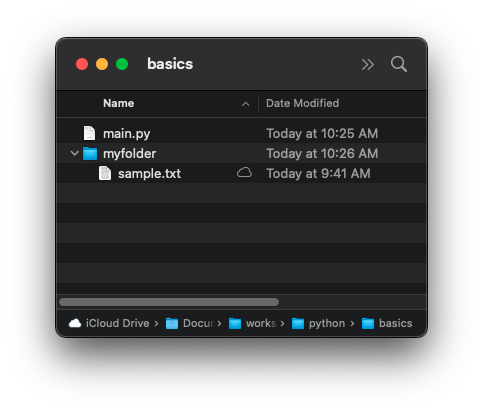
Python Program
import shutil
source = 'myfolder/sample.txt'
target = 'myfolder/sample_2.txt'
try :
shutil.copy(source, target)
print('File copied successfully.')
except:
print('Could not copy file.')
print('An exception occurred.')
Output
File copied successfully.
Copied File
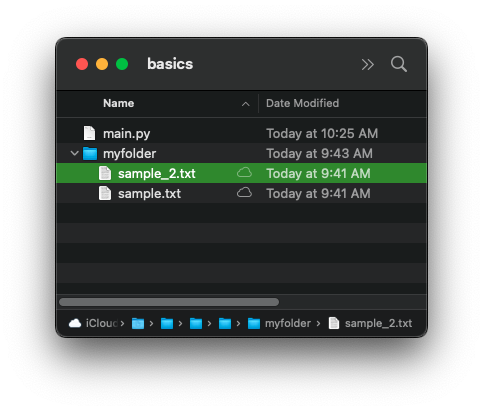
You may observe that the Date Modified is same as original file, since the meta of the original file is preserved while copying.
Summary
In this tutorial of Python Examples, we learned how to copy a file using shutil.copy() or shutil.copy2() function.