How to Reset Index of Pandas DataFrame? Examples
Pandas Reset Index of DataFrame
When you concatenate, sort, join or do some rearrangements with your DataFrame, the index gets shuffled or out of order.
To reset the index of a dataframe, you can use pandas.DataFrame.reset_index() method.
In this tutorial, you'll learn how to reset the index of a given DataFrame using DataFrame.reset_index() method.
Syntax of reset_index()
The syntax of DataFrame.reset_index() function is given below.
DataFrame.reset_index(level=None, drop=False, inplace=False, col_level=0, col_fill='')
To reset the index, pass the parameters drop=True and inplace=True.
Examples
1. Reset index of DataFrame using reset_index()
In this example, we will concatenate two dataframes and then use reset_index() to re-order the index of the dataframe.
Python Program
import pandas as pd
df_1 = pd.DataFrame(
[['Somu', 68, 84, 78, 96],
['Kiku', 74, 56, 88, 85],
['Ajit', 77, 73, 82, 87]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
df_2 = pd.DataFrame(
[['Amol', 72, 67, 91, 83],
['Lini', 78, 69, 87, 92]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
#concatenate dataframes
df = pd.concat([df_1, df_2])
#now the index is not in order
# reset index
df.reset_index(drop=True, inplace=True)
#print dataframe
print(df)
Explanation
- The program imports the
pandas
library, which is used to work with data in a tabular format. - Two DataFrames,
df_1
anddf_2
, are created using thepd.DataFrame()
function: df_1
: Contains data for three students with their scores in'physics'
,'chemistry'
,'algebra'
, and'calculus'
.df_2
: Contains data for two additional students with similar columns asdf_1
.- The
pd.concat()
function is used to concatenate the two DataFramesdf_1
anddf_2
vertically. The rows from both DataFrames are combined into one DataFrame. - After concatenation, the index values from both DataFrames are preserved, leading to non-sequential indices in the concatenated DataFrame.
- To reset the index and have it start from 0, the
reset_index()
method is called with the argumentdrop=True
, which removes the old index and assigns a new default sequential index. - The program then prints the final DataFrame,
df
, showing all rows with the reset index.
Output
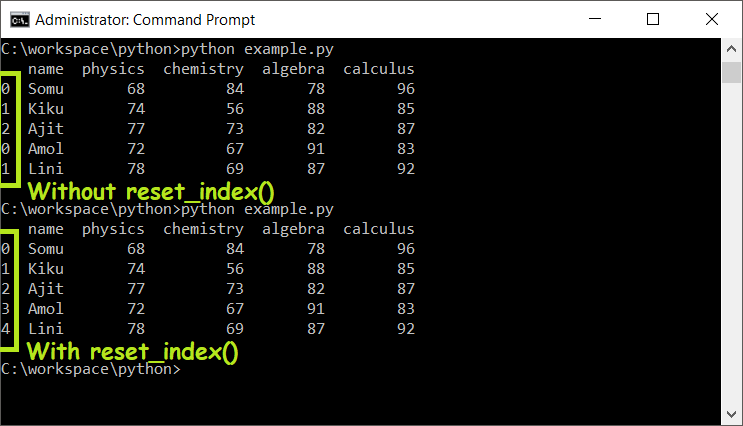
2. Reset index of DataFrame using concat()
You can reset the index using concat() function as well. Pass in the argument ignore_index=True
to the concat() function.
Python Program
import pandas as pd
df_1 = pd.DataFrame(
[['Somu', 68, 84, 78, 96],
['Kiku', 74, 56, 88, 85],
['Ajit', 77, 73, 82, 87]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
df_2 = pd.DataFrame(
[['Amol', 72, 67, 91, 83],
['Lini', 78, 69, 87, 92]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
#reset index while concatenating
df = pd.concat([df_1, df_2], ignore_index=True)
#print dataframe
print(df)
Explanation
- The program imports the
pandas
library, which is used for data manipulation and analysis. - Two DataFrames,
df_1
anddf_2
, are created using thepd.DataFrame()
function: df_1
: Contains data for three students with their scores in'physics'
,'chemistry'
,'algebra'
, and'calculus'
.df_2
: Contains data for two additional students with similar columns asdf_1
.- The
pd.concat()
function is used to concatenatedf_1
anddf_2
along the rows. The argumentignore_index=True
ensures that the indices are reset to a default sequential order, starting from 0, without preserving the original index values. - The resulting DataFrame,
df
, contains all the rows from bothdf_1
anddf_2
, with a new index assigned to each row. - The final DataFrame,
df
, is printed to show the combined data with the reset index.
Output
name physics chemistry algebra calculus
0 Somu 68 84 78 96
1 Kiku 74 56 88 85
2 Ajit 77 73 82 87
3 Amol 72 67 91 83
4 Lini 78 69 87 92
If you have only one dataframe whose index has to be reset, then just pass that dataframe in the list to the concat() function.
Python Program
import pandas as pd
df_1 = pd.DataFrame(
[['Somu', 68, 84, 78, 96],
['Kiku', 74, 56, 88, 85],
['Ajit', 77, 73, 82, 87]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
df_2 = pd.DataFrame(
[['Amol', 72, 67, 91, 83],
['Lini', 78, 69, 87, 92]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
#concatenate
df = pd.concat([df_1, df_2])
#now the index is not in order
#reset the index
df = pd.concat([df], ignore_index=True)
#print dataframe
print(df)
Video Tutorial - Reset Index of Pandas DataFrame
Summary
In this Pandas Tutorial, we learned how to resent index of Pandas DataFrame.