Deleting Columns in a Pandas DataFrame Using pop()
Pandas DataFrame.pop() - Delete Column
Pandas DataFrame.pop() function is used to delete a column from the DataFrame.
In this tutorial, we shall go through examples to learn how to use pop() to delete a column from Pandas DataFrame, with examples.
Examples
1. Deleting a column using pandas pop() function
In this example, we deleted a specific column, using column name, from the DataFrame with pop(). pandas pop() function updates the original dataframe. The data in the deleted column is lost.
Python Program
import pandas as pd
mydictionary = {'names': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]}
# Create DataFrame
df_marks = pd.DataFrame(mydictionary)
print('Original DataFrame\n--------------')
print(df_marks)
# Delete column whose name is 'algebra'
df_marks.pop('algebra')
print('\n\nDataFrame after deleting column\n--------------')
print(df_marks)
Output
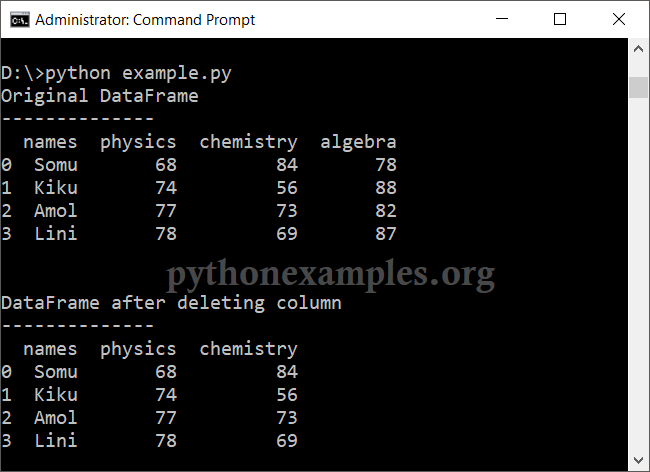
2. Deleting a non-existing column using pandas pop() function
In this example, we will try deleting a column that is not present in the DataFrame.
When you try to delete a non-existing column of DataFrame using pop(), the function pop() throws KeyError.
Python Program
import pandas as pd
mydictionary = {'names': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]}
# Create DataFrame
df_marks = pd.DataFrame(mydictionary)
print('Original DataFrame\n--------------')
print(df_marks)
# Delete column that is not present
df_marks.pop('geometry')
print('\n\nDataFrame after deleting column\n--------------')
print(df_marks)
Output
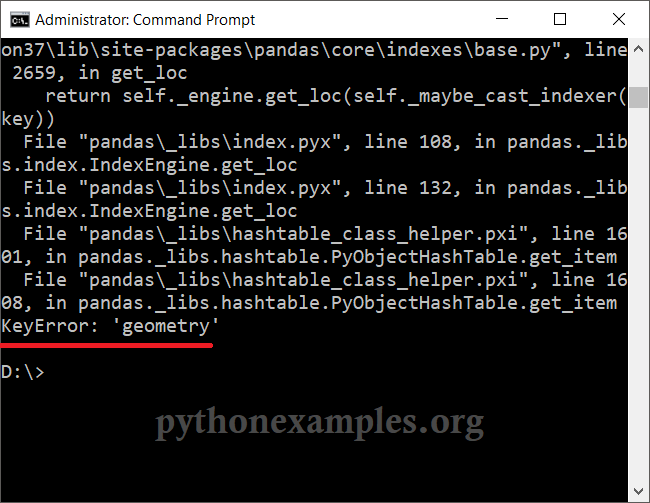
Summary
In this tutorial of Python Examples, we learned how to delete a column from DataFrame using pop() with the help of well detailed example programs.